Arduino Programming
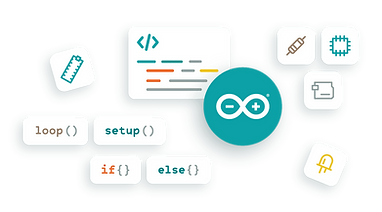
Introduction
​
Once you are done downloading and installing your Arduino IDE as we discussed in our previous tutorial, it is time to start writing our code (Arduino sketch). Here we will cover the essential fundamentals of Arduino Programming which can be divided into three main parts: structure, values (variables and constants), and functions.
What We'll Learn
​
What We'll Need
​
-
Computer running Arduino IDE.
-
Arduino Board (e.g. UNO).
-
Compartible USB cable.
Here, we will only focus on the basic compositions of Arduino programming to help beginners get started. You can visit the Arduino website to get the complete list of what we'll discuss in this tutorial.
Arduino program (sketch):
​
An Arduino sketch is a set of instructions that you create to carry out a particular task; in other words, a sketch is just a fancy name for a program. An Arduino sketch is divided into two main sections known as the structure of the sketch. These are the setup() and the loop() sections. We'll go into these later. Before we begin to write code, let's look at the programing syntax for Arduino.
Arduino programming syntax
What is Programming Syntax
Programming syntax is basically a set of rules that defines the combination of symbols that are considered to be correctly structured statements in that language.
​
Just as our conventional languages have rules we have to follow for them to make sense, programming languages also have rules to follow in order to be executed correctly.
​
As humans, we might sometimes get away with the wrong syntax in our conventional languages, such as omitting punctuations and grammatical errors because we may be smart enough to get out the intended meaning from a given data even though presented in not so correct format. With computers, this is not the case.
Computers operate with the garbage in, garbage out (GIGO) principle, meaning if what you give to your computer (program) is incorrect, the output also will not be the desired result (in most cases, you'll be stuck with an annoying error message with no output).
Arduino IDE sometimes helps us in pointing out errors and maybe where to locate them. On that note, the syntax for a particular programming language (Arduino in this case) must be followed strictly.
Some of the general syntax used in Arduino programming include the following elements:
​
Comments
A comment is a note of any length in a sketch, useful for adding notes, entering instructions, or any other miscellaneous details. When programming your Arduino or programming in any other language, it’s always a good practice to add comments regarding what your program is meant to do. These comments can prove useful later when you or any other person is revisiting a sketch.
​
In Arduino programming, there are two types of comments: single-line comments, and multi-line comments. To add a single line to your sketch, enter two forward slashes '//' and then your comment, as shown below:
​
...comment in Arduino IDE appears in grey color.
// Blink LED sketch by Jude Nkereuwem, created 05/05/20
The two forward slashes tell the IDE to ignore the text following when verifying a sketch (checking that the sketch is written properly without errors).
​
To add a multi-line comment to your sketch, enter the characters /* before your comment, then end the comment with the characters */, as shown in the example below:
/*
Arduino Blink LED sketch
by Jude Nkereuwem
created 05/05/20
*/
As discussed earlier, the /* and */ characters also tell the IDE to ignore the texts enclosed in them.
​
Semicolons ';'
Semicolons are to Arduino what period is to the English language. These are used at the end of a statement in the sketch. Forgetting to add a semicolon at the end of any statement will prompt an error notification. An example is shown with a variable declaration below:
​
int a = 100;
​
​
#define
We use this command to assign a variable to a constant. The syntax is as shown:
#define a 100
​
This tells the compiler to replace 'a' with 100 anywhere 'a' is called in the program. We do not use this command with an equal sign or add a semicolon after this command, as this will prompt an error message, as shown below:
​
#define a 100 // correct
#define a 100; // error
#define a = 100 // error
​
​
#include<>
With this command, we tell the Arduino to include additional library files in our sketch. This is a very useful command for Arduino, as it helps us add library files to our sketch for increased functionality (we'll look at libraries later in this course).
Just as with the #define command, we do not add semicolons after this command. The syntax for this command is as shown:
​
#include<library file>
​
​
Curly braces '{}'
Curly braces or brackets are an important part of Arduino language just as with C/C++ programming languages. The code within the braces is said to be a block that may be a function (a code to perform a specific task).
An open curly brace '{' must be followed by a closing curly brace '}'.
Arduino Keywords
​
Before we get to the text topic on our list, if you tried typing the commands we've discussed so far in your Arduino IDE, you must have noticed that some of the commands changed color as you were typing. This notifies you that these commands are keywords.
Keywords are those words already used by a particular language for a special purpose. This means you can't use these words again in your program to represent another purpose except the ones they were meant for.
For example, you can't declare a variable and name it '#include', you will be stuck with error messages.
Structure of an Arduino sketch
As was introduced earlier, a sketch has two main parts in its structure: these are setup() and loop().
​
The Setup Function
​
Syntax: void setup(){}
​
This section of your sketch contains a set of instructions for the Arduino to execute only once, each time the Arduino is turned on or the Reset button is pressed. This section is usually used to initialize added libraries and hardware pins. To create the setup function, add the lines of code shown below to your sketch. The Arduino IDE makes things even easier by adding this section automatically to your IDE text section when you open a new sketch file.
​
void setup()
{
//Enter your statement here to run once.
}
​
​
The Arduino IDE does not save your sketches automatically, so save your sketches as frequently as possible to avoid losing any of your work (click on the File menu then click on Save).
​
The Loop Function
Syntax: void loop(){}
​
This section of your sketch ('loop' as the name implies), contains a set of instructions for the Arduino to execute repeatedly, each time the Arduino is turned on or the Reset button is pressed. If you have a task you wish to perform repeatedly, this section of your sketch is the best place for the command.
​
void loop()
{
//Enter your statement here to run repeatedly.
}
​
The Arduino IDE automatically creates these two sections whenever you create a new sketch file.
Variables and Data Types
​
A variable such as in every other programming language is basically allocating a computer memory space for a specific purpose, such as storage of values. That is, you can allocate memory space, name it x for easy identification then assign the value 5 to it.
​
Types of variables
​
Local variable
Local variables are variables with local scope i.e. they can only be used within the code block where they are declared.
​
Global variable
Global variables are variables with global scope i.e. they can be used anywhere in the sketch. These are usually declared at the beginning part of the sketch (before the void setup function).
​
Data Types
​
Since Arduino programming is based on C/C++ syntax, you can not just store a value inside a memory location without indicating the type of the value you're storing. This brings us to Data Types. This simply refers to the type of particular data. For example; 5 is data of type integer, 5.3 is data of type float, and so on.
​
In our previous example, we created a memory location named x to store the value 5. We have to tell our compiler that the value 5 is an integer before the program can be executed correctly. The Arduino programing language has about 18 datatypes. We will look at the most commonly used ones in this section. The rest can be found on the Arduino website.
​
Syntax: Datatype Variable name = Data
​
Integers (int)
Integers are the data type that can store numbers. The int data type has at least 4 bytes in size. To create a variable to store an integer in your sketch, begin your variable with the keyword 'int', as shown below:
​
int myInt = 5;
Characters (char)
This helps us to store a single character. The char data type has at least an 8-bit size. The character must be in single quotes. The syntax is as shown below;
​
char myChar = 'A';
​
Floating Point Numbers (float)
It helps to store decimal numbers. Floats are used to approximate the analog and continuous values due to their greater resolution when compared to integers. Float ranges from -3.4028235E+38 to 3.4028235E+38 and is generally limited to six or seven decimal places. It has 32-bit storage space. You can mix integers and float numbers in your calculations. Syntax below:
​
float myFloat = 1.164;
​
Boolean (bool)
Sometimes you need to record whether something is in either of only two states, such as on or off, or hot or cold. The Boolean variable is the computer "bit" whose value can only be zero (0, false) or one (1, true). It occupies one byte. Like any other variable, we need to declare it in order to use it:
​
boolean hot = true;
​
It’s simple to use Boolean variables to make decisions using an if test structure. Because Boolean comparisons can either be true or false, they work well with the comparison operators '!=' and '=='.
​
Arrays
An array is a group of variables or values grouped together so that they can be referenced as a whole. This is very useful when dealing with lots of related data. Each item in an array is called an element. We count the elements in an array beginning from left to right and starting from 0; the first element of the array is always at index 0, and the size of the array is a total number of elements -1.
​
An array to store a particular datatype should be declared with a keyword for such datatype. For example, let's declare an array of integers with six elements below:
​
int a[6];
​
We can also insert values when defining the array. Using this method, we do not need to define the array size, the size is deduced based on the number of elements set by the values inside the curly brackets '{}':
​
int a[]={1,2,3,4,5,6};
​
String
A string of text is a combination of characters. In Arduino, strings can be represented in two ways. You can use the string data type or you can make a string out of an array of type cha and null terminate it. Here we will look at the latter option. Syntax below:
​
char myString[8] = {'a','r','d','u','n','o','\0'};
​
Above, we created an array to store the characters of the text 'arduino' as individual elements then terminate it with a null character. Generally, strings are terminated with a null character (ASCII code 0). This allows functions (like Serial.print()) to tell where the end of a string is. Otherwise, they would continue reading subsequent bytes of memory that aren't actually part of the string. This means that your string needs to have space for one more character than the text you want it to contain.
​
Byte
A byte stores an 8-bit unsigned number, from 0 to 255. Syntax below:
​
byte b = B10010; // "B" is the binary formatter (B10010 = 18 decimal)
Operators
​
Boolean Operators
We can use various operators to make decisions about two or more Boolean variables or other states. These include the operators not (!), and (&&), and or (||).
​
The 'not' Operator (!)
The 'not' operator is denoted by the use of an exclamation mark (!). This operator is used as an abbreviation for checking whether something is not true. Here’s an example:
​
if ( !raining )
{
// it is not raining (raining == false)
}
​
The 'and' Operator (&&)
The logical 'and' operator is denoted by &&. Using and helps reduce the number of separate if tests. Here’s an example:
​
if ((raining == true) && (!summer))
{
/*
It is raining and not summer
(raining == true and summer == false)
*/
}
​
The 'or' Operator (||)
The logical 'or' operator is denoted by ||. Using 'or' is very simple; here’s an example:
​
if (( raining == true ) || ( summer == true ))
{
// it is either raining or summer
}
Arithmetic Operators
​
We can perform all the basic mathematical operations in the Arduino programming language using arithmetic operators.
​
addition: '+'
Used to add two or more values.
​
Syntax: value1 + value2;
​
Example:
​
int a = 2
int b = 5
c = a+b; // c equal to 7
​
subtraction: '-'
Used to subtract one value from another.
​
Syntax: value1 - value2;
​
Example:
​
int a = 2
int b = 5
c = b-a; // c equal to 3
​
multiplication: '*'
Used to multiply two or more values.
​
Syntax: value1 * value2;
​
Example:
​
int a = 2
int b = 5
c = a*b; // c equal to 10
​
division: '/'
Used to divide two values.
​
Syntax: quotient = divider / divisor;
​
Example:
​
int a = 10
int b = 2
c = a/b; // c equal to 5
Comparison Operators
​
In addition to the comparison operators discussed above, we can also use the following to compare numbers or numerical variables in an Arduino program:
​
< less than
> greater than
== equal to
!= not equal to
<= less than or equal to
>= greater than or equal to
​
​
Compound Operators
​
++ (increment)
-- (decrement)
+= (compound addition)
*= (compound multiplication)
/= (compound division)
%= (compound modulo)
&= (compound bitwise and)
|= (compound bitwise or)
Control statements
​
This commands allows you to add different controls to your program. Examples of this types of control commands are discussed below:
​
'if' statement
if, which is used in conjunction with a comparison operator (==, !=, <, >), tests whether a certain condition has been reached, such as an input being above a certain number.
​
Syntax:
if (someVariable > 50)
{
//do something here
}
​
Example:
if(x > 50)
{
digitalWrite(13, HIGH);
}
​
'if...else' statement
if/else allows greater control over the flow of code than the basic if statement, by allowing multiple tests to be grouped together. For example, an analog input could be tested and one action taken if the input was less than 500, and another action taken if the input was 500 or greater.
​
Example:
if (pinFiveInput < 500)
{
// action A
}
else
{
// action B
}
​
'for' statement
​
Syntax: for (starting condition; stopping condition; incrementing/decrementing condition){}
​
Example:
for(i=0; i<=10; i++)
{
digitalWrite(13, HIGH);
}
​
In the above sketch, i is initialized as 0, it stops when i is incremented (i++) to 10.
​
Arguments: There are three arguments inside the 'for' statement; the starting, stopping and the changing conditions.
​
Starting condition: This is where you initialize the variable and assign the starting number to that variable.
​
Stopping condition: This statement runs the code until the variable satisfies the specified condition.
​
Changing condition: This specifies how to increment or decrement the variable for upcoming iterations.
​
'while' statement
You can use the while() statements in a sketch to repeat instructions, as long as (while) given condition is true. The condition is always tested before the code in the while() statement is executed.
​
Syntax: while(stopping condition){incrementing/decrementing statement)
​
Arguments: Stopping condition.
​
There must be an increment/decrement statement within the 'while' block. Also, you need to initialize the variable before calling the 'while' statement.
​
Example:
int a = 0;
while ( a < 10 )
{
a = a + 1;
delay(1000);
}
​
This sketch starts with the variable a set to 0. It then adds 1 to the value of a, waits 1 second (delay(1000)), and then repeats the process until a has a value of 10. Once a is equal to 10, the comparison in the while statement is false; therefore, the Arduino will continue on with the sketch after the while loop brackets.
'do-while' statement
In contrast to while, the do-while() structure places the test after the code within the do-while statement is executed.
​
Syntax: do{}while(stopping condition)
​
Example:
int a = 0;
do
{
delay(1000);
a = a + 1;
} while ( a < 100 );
​
In this case, the code between the curly brackets will execute before the conditions of the test (while ( a < 100 )) have been checked. As a result, even if the conditions are not met, the loop will run once. You’ll decide whether to use a while or a do-while function when designing your particular project.
​
'break' statement
This statement is used to break the loop when a given condition is satisfied. The program will exit the loop and continue executing the rest of the code.
​
Syntax: break
​
Example:
int a;
while(True){
a = digitalRead(3); //set pin 3 as input
if(a==1){ //check if pin 3 == 1
break; //break if pin 3 == 1
}
}
'return' statement
This is used to return a value to the variable. This is usually used in a function. The value may be of any dat types, but it should be specified at the function declaration.
​
Syntax: return value
​
Example:
int value(int a){
if(a>50){
return a-50;
}
else{
return a;
}
}
​
void loop(){
int a;
a = value(analogRead(3));
Serial.println(a);
}
​
The above sketch tells the compiler: if the values read at pin3 is not equal to
50, return to the function. Then print the original value of a - 50 till a reaches 50.
​
'continue' statement
This tells the compiler to skip the next instruction when the given condition is satisfied.
​
Syntax: continue
​
Example:
for(int i=0;i<100;i++){ //checks if i is even or not
if(i%2==0){
continue; //skip the statement below if i == even number
}
analogWrite(3, i)
delay(50);
}
goto statement
Points the compiler to the specific instruction to be executed if a condition is satisfied.
​
Syntax: goto
​
Example:
int a;
while(1){
if(analogRead(3)>250){
goto action; //pass to action if analog reading is > 250
}
}
​
action:
digitalWrite(3, HIGH);
​
Creating Custom Functions in Arduino
​
A function consists of a set of instructions that can be used anywhere in our sketches to perform a specific task. Although there are many inbuilt functions in the Arduino language, sometimes you may not one that suit your specific needs, or you might just want to run part of a sketch repeatedly and you don't want to rewrite the code each time you want to use it which is also a waste of memory.
​
Creating a Function to repeat an action
You can write simple functions to repeat actions when needed. For example, in our sketch below, we will need a command to control the built-in LED on our Arduino board (to turn ON and OFF twice), and we will write a function (blinkLED) to handle that.
​
#define LED 13
#define del 200
​
void setup()
{
pinMode(LED, OUTPUT);
}
​
void blinkLED(){
digitalWrite(LED, HIGH);
delay(del);
digitalWrite(LED, LOW);
delay(del);
digitalWrite(LED, HIGH);
delay(del);
digitalWrite(LED, LOW);
delay(del);
}
​
void loop(){
blinkLED();
delay(1000);
}
When the blinkLED() function is called in void loop(), the Arduino will run the commands within the blinkLED() function.
Creating a Function to return a value
In addition to creating functions that accepts values as parameters, you can also create functions that return a value. Most of the functions we've seen up to this point beging with void; meaning the function doesn't return anything, i.e. the function return value is void. Now, let's crete functions that return actual values. For example, let's create a function that converts degree Celsius to Farenheit:
​
float convertTemp(float celsius)
{
float fahrenheit = 0;
fahrenheit = (1.8 * celsius) + 32;
return fahrenheit;
}
​
First, we define the function name (convertTemp), which will return variable type (float); you can pass in any variable. For example, if we want to convert 50 degrees Celsius to Farenheit and store the result in a flost variable called tempf, then we will call the function as below:
​
tempf = convertTemp(50);
​
This would place 50 into the convertTemp variable celsius and use it in the calculation of fahrenheit = (1.8 * celsius) + 32 in the convertTemp function. The result is then returned into the variable tempf with the convertTemp line return fahrenheit.