Raspberry Pi Pico: Analog Signal
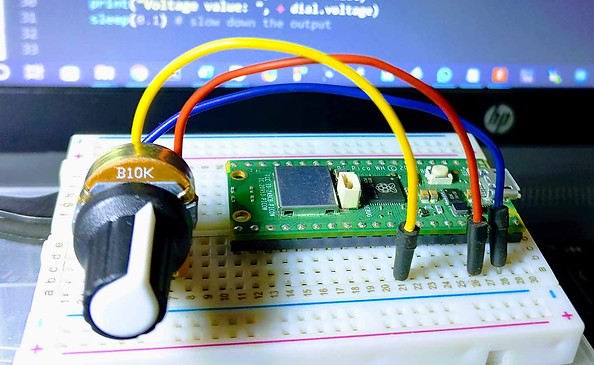
Introduction
​
Analog signals are continuous signals that vary smoothly over time and can take on any value within a certain range. These signals represent physical quantities such as voltage, current, temperature, pressure, or sound waves in the real world.
​
Analog signals are used in various applications such as audio processing, telecommunications, instrumentation, and control systems. They are often converted into digital signals for processing by computers or digital devices, a process known as analog-to-digital conversion (ADC), but they remain prevalent in many aspects of technology and everyday life.
​
The Raspberry Pi Pico has four 12-bit ADC channels dedicated to analog input. One of these four pins is connected to the internal temperature sensor. The remaining ADCs are located at GPIO26, GPIO27, and GPIO28 as ADC0, ADC1 and ADC2,
respectively.
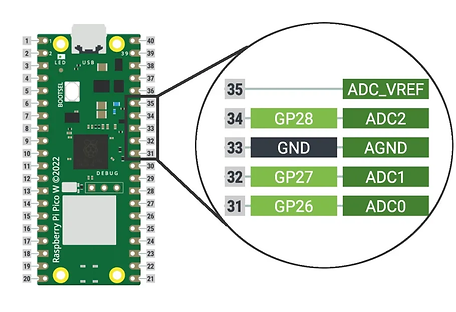
As mentioned earlier, the ADC channels are 12-bit, however, when we program the Pico with MicroPython, we get a 16-bit resolution. This is because, in the MicroPython ADC library, they have scaled the 12-bit resolution into a 16-bit resolution, which is why we will have a maximum ADC value of 65535 (i.e. 2^16) instead of 4096 (i.e. 2^12).
​
​
Potentiometers
​
A potentiometer is a resistor that allows you to change the value of the resistance. They are often just called pots.
​
Turning the dial on the top of the potentiometer will change the resistance of the potentiometer, which the Raspberry Pi Pico can then read.​
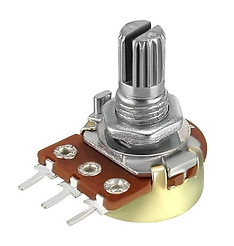
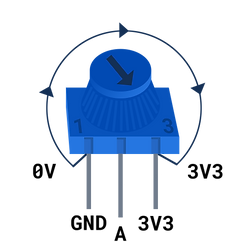
We'll be using a potentiometer to provide our analog input. First, let's assemble our hardware.
​
Hardware components
-
Raspberry Pi Pico
-
Breadboard
-
Potentiometer
-
Male-Male jumper wires
-
LED
​
Assemble Hardware
-
Fix your Pico to the breadboard.
-
Fix the potentiometer also to the breadboard. Connect the center pin of the potentiometer to GPIO26 (ADC0) of the Pico, then one end of the potentiometer to any GND pin of the Pico, and the other pin a 3.3v pin of the Pico with jumper wires.
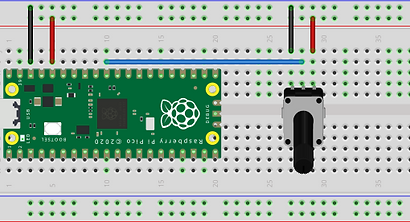.png)
We'll read the potentiometer values first and display it on Thonny using MicroPython, with the few lines of code below:
​
from machine import ADC, Pin
import time
​
adc = ADC(Pin(26))
​
while True:
print(adc.read_u16())
time.sleep(1)
​
Run your code. Turn your potentiometer dial, yo should see values (approximately 0 and 65025) displaying in the shell section of the Thonny window.
Control LED brightness with PWM
​
Pulse width modulation allows us to give analog behaviors to digital devices, such as LEDs. This means that rather than an LED being simply on or off, you can control its brightness.
​
Let's control the brightness level of our LED using PWM signals from the Raspberry Pi Pico with code below:
​
from machine import Pin, PWM
from time import sleep
​
pwm = PWM(Pin(15))
pwm.freq(1000)
​
while True:
for duty in range(65025):
pwm.duty_u16(duty)
sleep(0.0001)
for duty in range(65025, 0, -1):
pwm.duty_u16(duty)
sleep(0.0001)
Save and run the file. You should see the LED pulse bright and dim, in a continuous cycle.
​
The frequency (pwm.freq) tells Raspberry Pi Pico how often to switch the power between on and off for the LED.
​
The duty cycle tells the LED for how long it should be on each time. For Raspberry Pi Pico in MicroPython, this can range from 0 to 65025. 65025 would be 100% of the time, so the LED would stay bright. A value of around 32512 would indicate that it should be on for half the time.
​
You can play with the pwm.freq() values and the pwm.duty_u16 values, as well as the length of time for the sleep, to get a feel for how you can adjust the brightness and pace of the pulsing LED.
Control LED brightness with Potentiometer
.png)
Let's now use these values from the potentiometer to control the duty cycle for PWM on the LED.
Use the code below:
​
from machine import Pin, PWM, ADC
​
pwm = PWM(Pin(15))
adc = ADC(Pin(26))
​
while True:
duty = adc.read_u16()
pwm.duty_u16(duty)
Once you run the code, tune the dial on the potentiometer to control the LED's brightness.