Raspberry Pi GPIO Pins
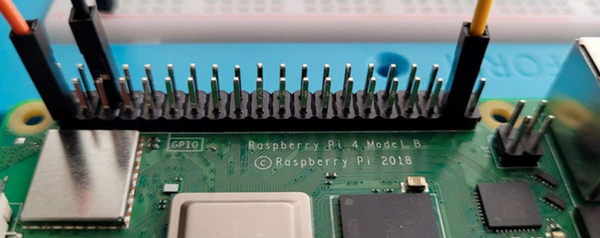
Course Outline​​
-
Introduction
-
Pin Numbering
-
Pin Types
-
GPIO Pin Functions​
-
Interfacing Digital peripherals to Pi via GPIO​
-
Interfacing Analog peripherals to Pi via GPIO​​
1. GPIO Pins
​
Raspberry Pi GPIO (General Purpose Input/Output) pins are versatile digital pins on the Raspberry Pi board that can be used for various purposes, including input, output, PWM, I2C, SPI, UART, and more. These pins are crucial for interfacing with other components like sensors, motors, LEDs, and other electronic devices. We refer to this as physical computing. Raspberry Pi's GPIO pins are highly flexible and can be configured for various functions, making them suitable for a wide range of electronic projects.
2. Pin Numbering:
​
-
Physical Pin Numbering: Refers to the actual pin numbers on the Raspberry Pi's GPIO header. The Raspberry Pi typically has a 40-pin header (on models like Raspberry Pi 5, 4, 3, and 2).
​
-
Broadcom (BCM) Numbering: Refers to the GPIO pin numbers as defined by the Broadcom SoC (System on Chip) used in the Raspberry Pi. This numbering is commonly used in software when controlling the GPIOs.
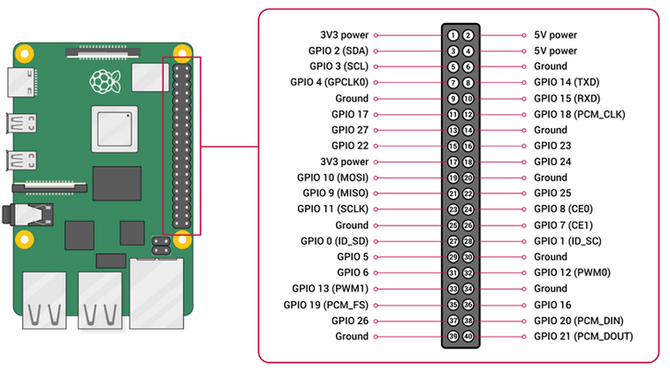
Raspberry Pi GPIO Pin Diagram
3. Pin Types:​
1. P​ower Pins:
​​​
-
3.3V Pins (Pin 1, Pin 17): These provide a constant 3.3V output, used to power small components that require 3.3V.
-
5V Pins (Pin 2, Pin 4): These provide a constant 5V output, used to power components that require 5V.
-
GND (Ground) Pins: These are connected to the ground of the system, used as a reference point for voltage and as a return path for current.
​
2. GPIO Pins:
-
General I/O: The basic use of GPIO pins is as input or output pins. When set as an output, the pin can be set high (3.3V) or low (0V). When set as input, the pin can read high or low states.
-
Special Functions: Some GPIO pins have alternate functions such as PWM (Pulse Width Modulation), I2C (Inter-Integrated Circuit), SPI (Serial Peripheral Interface), and UART (Universal Asynchronous Receiver-Transmitter).
​
​​
​
4. GPIO Pin Functions:
​
1. Digital Input/Output: Pins can be configured to read digital signals (high or low) or output digital signals.
​
​2. PWM Output GPIO18 (Pin 12), GPIO12 (Pin 32), GPIO13 (Pin 33): Some GPIO pins can output PWM signals, which are useful for controlling devices like servos or LEDs with varying brightness.
​​
​3. I2C Interface GPIO2 (Pin 3) and GPIO3 (Pin 5): Pins dedicated to the I2C communication protocol, typically used for connecting sensors and other peripherals.
-
SDA (Data Line): Used for transmitting data.
-
SCL (Clock Line): Used for synchronizing data transmission.
4. SPI Interface GPIO10 (Pin 19), GPIO9 (Pin 21), GPIO11 (Pin 23): Used for high-speed communication with peripherals like ADCs, DACs, and displays.
-
MOSI (Master Out Slave In): Data sent from the master to the slave.
-
MISO (Master In Slave Out): Data sent from the slave to the master.
-
SCLK (Serial Clock): Clock signal generated by the master.
-
CE (Chip Enable): Selects the slave device.
5. UART Interface GPIO14 (Pin 8) and GPIO15 (Pin 10): Serial communication pins used for transmitting (TX) and receiving (RX) data.
6. Ground (GND): Pins used to establish a reference voltage and return path for the circuit.
5. Interfacing Digital Peripherals to Pi via GPIO
As we saw earlier, the I/O in GPIO stands for Input and Output, respectively, meaning that for every component connected to the Pi, you either read signals from it (Input) or send signals to it (Output).
On a broader scale, the signals involved in these I/O operations can be classified into two categories: Digital and Analog signals.
​​
A. Digital Input Operations on Raspberry Pi
​​
Reading Digital Signals: You can configure a GPIO pin as an input to read digital signals. For example, you can connect a push button to a GPIO pin, and the Raspberry Pi can detect when the button is pressed (HIGH) or not pressed (LOW).
Component list:
-
Raspberry Pi 4 or 5
-
Breadboard
-
Push button
-
Jumper wires (2 Male – Female and 1 Male – Male)
​
Connect the circuit as shown below:
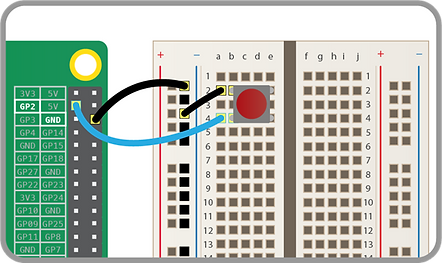
Raspberry Pi with Push button
Python code: button.py
from gpiozero import Button
button = Button(2)
button.wait_for_press()
print('You pushed me')
B. Digital Output Operations on Raspberry Pi
​
Controlling Digital Devices: You can set a GPIO pin as an output to control digital devices like LEDs, relays, or buzzers. The Raspberry Pi can switch the pin state to HIGH (3.3V) or LOW (0V) to turn the device on or off.
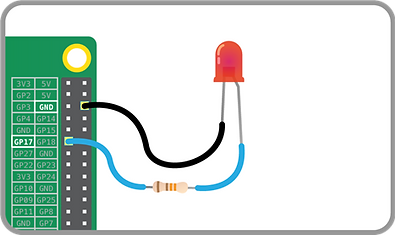
Raspberry Pi with LED
Python code: led.py
​
from gpiozero import LED
led = LED(17)
​
while True:
led.on() #This command will keep your LED on
led.off() #This command will keep your LED off
To blink your LED, you can add the sleep() function to turn on the LED, pause for some time, then turn it off. Having this inside a loop will keep your LED blinking.
Python code: blink_led.py
​
from gpiozero import LED
from time import sleep
led = LED(17)
​
while True:
led.on() #LED on
sleep(1) #pause for 1 second
led.off() #LED off
sleep(1) #pause for 1 second
6. Interfacing Analog Peripherals to Pi via GPIO
​
Unlike some microcontrollers (e.g., Arduino), the Raspberry Pi does not have built-in analog-to-digital (ADC) or digital-to-analog (DAC) converters. This means that the Raspberry Pi cannot natively read analog signals directly from sensors like temperature or gas sensors, nor can it output true analog signals.
A. Reading Analog Signals: External ADC:
​
To read analog signals, you need an external ADC chip, such as the ADS1015/ADS1115. The ADS1x15 can be connected to the Raspberry Pi via the I2C interface, allowing the Pi to read analog signals from sensors.
The ADS1115 breakout board is a high-resolution analog-to-digital converter (ADC) that can be used in conjunction with single-board computers such as the Raspberry Pi.
By incorporating the ADS1115 module, which boasts a 16-bit ADC resolution, the Raspberry Pi’s ability to handle analog inputs can be vastly expanded. With the ADS1115, the smallest voltage that can be measured is 5V / 65536 = 0.000076V (76uV), offering enhanced precision for your projects.
​
ADS1115/1015 External ADC
​
The ADS1115 (16-bit) and ADS1015 (12-bit) 4-channel breakout boards are perfect for adding high-resolution analog-to-digital conversion to any microprocessor-based project. These boards can run with power and logic signals between 2V to 5V, so they can be compatible with all common 3.3v and 5v processors. As many as four boards can be controlled from the same 2-wire I2C bus, giving you up to 16 single-ended or 8 differential channels.
​
These two boards are very similar, meaning they are connected the same way and even function with the same library. They differ only in resolution and speed. The ADS1115 has a higher resolution and the ADS1015 has a higher sample rate.
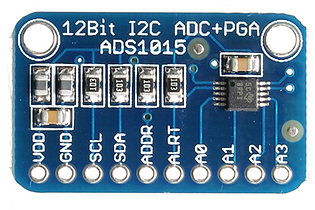
ADS1015 ADC
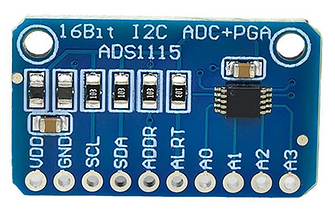
ADS1115 ADC
You can also make use of this two-in-one ADS1015/ADS1115 module then edit your code to select which resolution you wish to make use of.
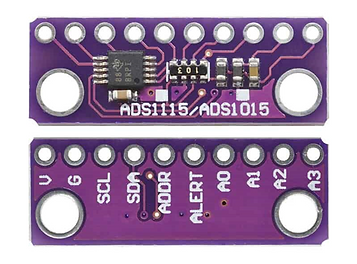
ADS1015/1115
Interfacing ADS1115 Module with Raspberry Pi
​
Interfacing an ADS1115 module with a Raspberry Pi 5 is straightforward, as both devices use the I2C communication protocol. Follow the circuit below to make your circuit connections.
Components:
-
Raspberry Pi
-
ADS1115
-
Breadboard
-
Male – Female jumper wires (4)
-
Male – Male jumper wires (5)
-
Potentiometer (analog sensor)
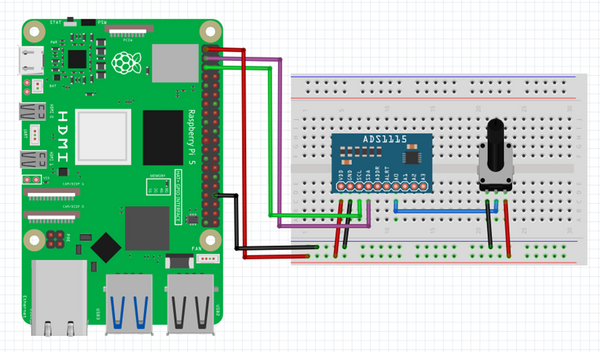
Raspberry Pi with Potentiometer
ADS1115 Python Library Installation
​
Before moving to the Python programming part, we need to install ADS1115 Library on Raspberry Pi.
Open a terminal window on your Raspberry Pi. Run the following command to install the ADS1x15 Python library.
sudo pip3 install adafruit-circuitpython-ads1x15
Below is a Python Code to interface ADS1115 16-bit ADC Module with Raspberry Pi. The code will read analog values from Pin A0 fed using the potentiometer.
Python code: ADS1115.py
​
import board
import busio
import adafruit_ads1x15.ads1115 as ADS
from adafruit_ads1x15.analog_in import AnalogIn
import time
​
i2c = busio.I2C(board.SCL, board.SDA) # Initialize the I2C interface
ads = ADS.ADS1115(i2c) # Create an ADS1115 object
​
# Define the analog input channels
channel0 = AnalogIn(ads, ADS.P0)
channel1 = AnalogIn(ads, ADS.P1)
channel2 = AnalogIn(ads, ADS.P2)
channel3 = AnalogIn(ads, ADS.P3)
# Loop to read the analog inputs continuously
while True:
print("Analog Value0: ", channel0.value, "Voltage0: ", channel0.voltage)
print("Analog Value1: ", channel1.value, "Voltage1: ", channel1.voltage)
print("Analog Value2: ", channel2.value, "Voltage2: ", channel2.voltage)
print("Analog Value3: ", channel3.value, "Voltage3: ", channel3.voltage)
time.sleep(1) # Delay for 1 second
​​
B. Analog Output (PWM) on Raspberry Pi:
​
PWM (Pulse Width Modulation): While the Raspberry Pi doesn't have a true DAC for analog output, it can simulate analog signals using PWM. PWM rapidly switches a GPIO pin between HIGH and LOW states, and by varying the duty cycle (the ratio of time the signal is HIGH vs. LOW), you can simulate a varying voltage.
To generate PWM (Pulse Width Modulation) output on the Raspberry Pi 5 using the GPIO Zero library, you can control devices like LEDs, motors, or servos by varying the duty cycle of the signal.
Here’s how you can create a simple PWM control to adjust the brightness of an LED:
Python code
​
from gpiozero import PWMLED
from time import sleep
​
# Define the GPIO pin number where the LED is connected (e.g., GPIO 18)
led = PWMLED(18)
​
# Increase and decrease brightness
while True:
for brightness in range(0, 101):
#Brightness value must be between 0 (off) and 1 (full brightness)
led.value = brightness / 100
sleep(0.01)
for brightness in range(100, -1, -1):
led.value = brightness / 100
sleep(0.01)