Raspberry Pi with DHT11/22
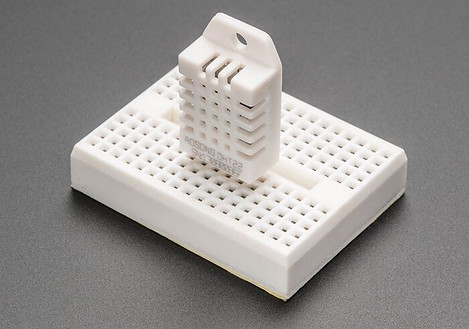
Introduction
​
In this lesson, you will learn how to connect and read data from a DHT11/22 temperature and humidity sensor using a Raspberry Pi.
​
Some (analog) sensors can work with the Raspberry Pi without needing external ADC – through the help of software libraries. An example of such sensors is a DHT sensor.
​
A DHT sensor (Digital Humidity and Temperature sensor) is commonly used for measuring temperature and humidity in various applications. There are two popular versions:​
-
DHT11 and DHT22
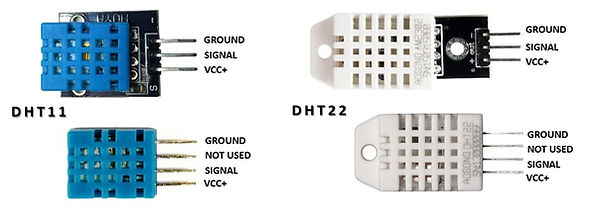
DHT11/22 Pinout
​Needed Components:
​
-
Raspberry Pi 5
-
DHT22 or DHT11
-
Breadboard
-
Jumper wires
-
10k ohms resistor
​
​
Both sensors communicate via a single-wire protocol, making them easy to interface with microcontrollers like the Arduino and Raspberry Pi.
Circuit Diagram

DHT22 Sensor module connected to Pin21 of Raspberry Pi 5
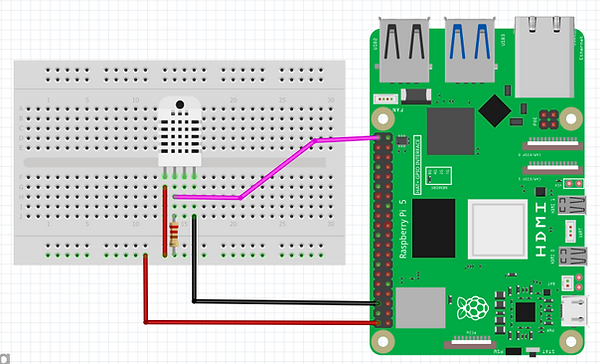
DHT22 Sensor (with 10K resistor) connected to Raspberry Pi 5
DHT22 Programming
​
Now install the DTOVERLAY DHT22 or DHT11 depending on the model you have (we will be using GPIO21 in this example):
​
-
Open Raspberry Pi Terminal and type: type “sudo nano /boot/firmware/config.txt”
-
Navigate down using the “down arrow button” to where you have [All]
-
Just after the [ALL] add this line: “dtoverlay=dht22,gpiopin=21” then click “Enter”
-
Press CTRL+X then type “yes” to save, then click “Enter”
-
Type “sudo reboot” then “Enter” to reboot your Pi and activate changes.
Once your Pi is back up, write the following Python code to read values from your dht22 sensor:
​
dht22.py code:
​
#!/bin/python3
import time
device0 = "/sys/bus/iio/devices/iio:device0"
​
#function to read the first line and return integer
def readFirstLine(filename):
try:
f = open(filename,"rt")
value = int(f.readline())
f.close()
return True, value
except ValueError:
f.close()
return False,-1
except OSError:
return False,0
​
try:
while True:
Flag, Temperature = readFirstLine(device0+"/in_temp_input")
print("Temperature:",end="")
if Flag:
print(Temperature // 1000,"\u2103",end="\t")
else:
print("N.A.",end="\t")
Flag, Humidity = readFirstLine(device0+"/
in_humidityrelative_input")
print("Humidity:",end="")
if Flag:
print(Humidity // 1000,"%")
else:
print("N.A.")
time.sleep(2.0)
except KeyboardInterrupt:
pass