ESP32 with OLED using MicroPython
OLED Display
​
An organic light-emitting diode (OLED or organic LED) is a flat light-emitting technology that places a series of thin organic films between two conductors. When an electrical current is applied, a bright light is emitted, OLEDs are emissive displays that do not require a backlight and so are thinner and more efficient than LCD displays (which do require a white backlight).
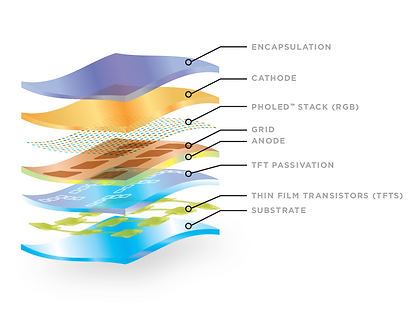
OLED Structure
OLEDs are used to create digital displays in devices such as television screens, computer monitors, and portable systems such as smartphones and handheld game consoles. OLED displays also provide the best image quality and can be made transparent, flexible, foldable and even rollable and stretchable in the future.
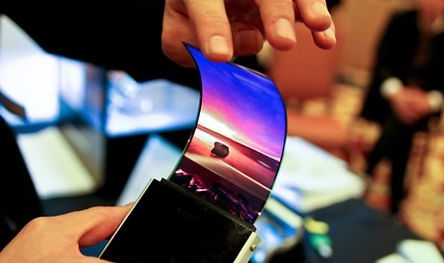
Since OLED display works without a backlight and is thinner in size, it makes it suitable for portable embedded devices and also saves energy which is a major factor in embedded system development. The OLED displays can vary in size, shape, and color, but they are primarily programmed in a similar way.
​
In our previous tutorial, we looked at how to display tests from the Pico using an LCD screen, here, we will be looking at how to interface an OLED display and how to display certain things.
SSD1306 OLED Display
​
Before we get to the main gist of this tutorial, let's look at the OLED display we'll be using. There are several types of OLED displays available in the market, for this tutorial, we'll be using an SSD1306 0.96-inch I2C OLED display. This display with the help of the SSD1306 controller uses I2C protocol to communicate with the microcontroller (although SPI can also be used in other modules). It also has 128x64 pixels.
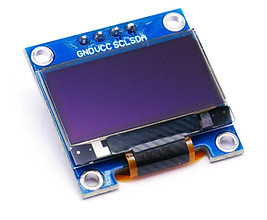
SSD1306 0.96 inch OLED
The SSD1306 OLED comes with four pins labeled VCC, GND, SCL, and SDA respectively. The VCC (3.3v-5v) and GND pins are for powering the display module, while SCL and SDA are for I2C communication with the microcontroller.
SSD1306 OLED Specifications
Interfacing OLED to ESP32
​
Hardware Needed
-
ESP32
-
SSD1306 0.96-inch 12C OLED display
-
Solderless breadboard
-
Male-Female jumper wires (x4)
OLED Wiring with ESP32
Layout
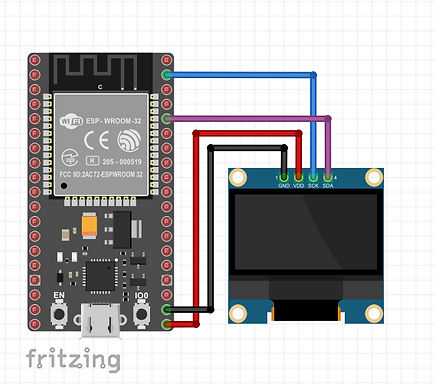.png)
Programming
SSD1306 OLED MicroPython Library
Before we continue, we'll need to install the SSD1306 OLED MicroPython Library in Thonny:
-
Plug in your Pico to your computer
-
Open Thonny IDE.
-
Click on Tools > Manage Packages. (This should open the Thonny Package Manager)
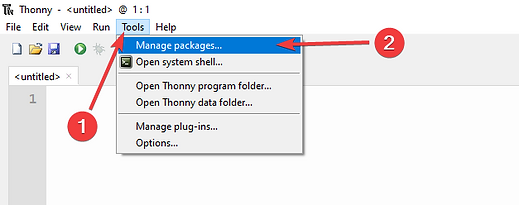
-
In the search bar: type "ssd1306" then click 'Search on PyPi' (a new page should appear with different options)
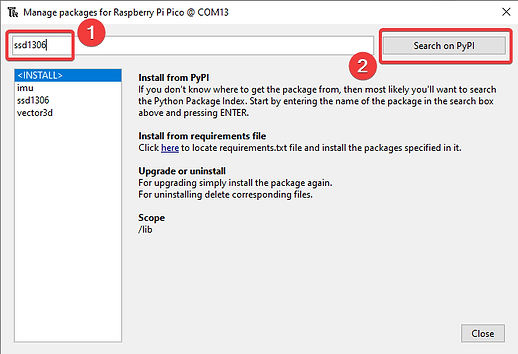
-
Click on "micropython-ssd1306" (a new page should pop up), the click on "Install"
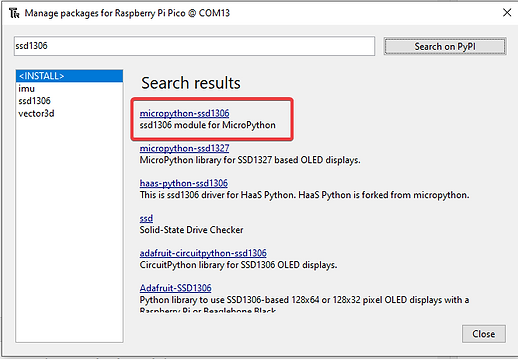
-
Click on "Close" after installation is complete. We are now set to program our OLED.
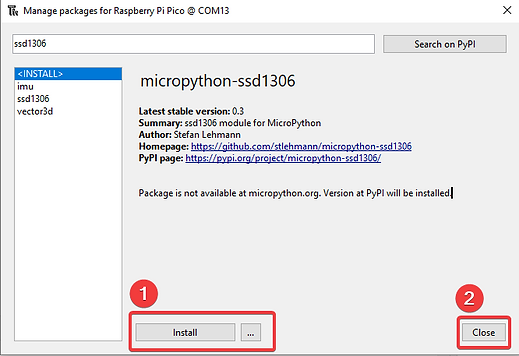
Code
from machine import SoftI2C, Pin
from ssd1306 import SSD1306_I2C
​
i2c = SoftI2C(scl=Pin(22), sda=Pin(21), freq=400000)
oled = SSD1306_I2C(128, 64, i2c)
​
oled.text(" Welcome to", 0, 16)
oled.text(" Placidlearn", 0, 30)
oled.show()
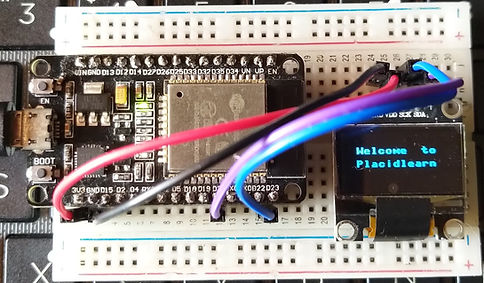
How Code works
​
Importing I2C and OLED Library
​
from machine import Pin, SoftI2C
from ssd1306 import SSD1306_I2C
​
We start by importing the Pins and SoftI2C classes from the machine module.
Next, we'll import the SSD1306 I2C library we previously installed.
Initializing OLED
​
i2c = SoftI2C(scl=Pin(22), sda=Pin(21), freq=400000)
​
Next, we'll initialize the SoftI2C() method which takes in three parameters. The first two parameters specify the I2C GPIO pins for SDA and SCL, which in this case are GPIO 21 and GPIO 22 respectively. Finally, we specify the frequency of communication between OLED and ESP32, we choose 400000 in this case. This information is saved in the i2c object.
​
oled = SSD1306_I2C(128, 64, i2c)
​
Next, we create an object 'oled' of SSD1306_I2C which uses the width, height, and i2c object as parameters: our OLED for this tutorial is 128X64 (width = 128, height = 64)
​
Displaying text on OLED
To display text on the OLED, we'll use the text() function from the 'oled' object previously initialized. The text() function takes three arguments.
​
oled.text(" Welcome to", 0, 16)
oled.text(" Placidlearn", 0, 30)
​
-
The first argument is the text we wish to display (e.g "Welcome!")
-
The second argument is the column you wish your first character to appear on.
-
Then lastly, the row you wish your text to appear.
​
If you are displaying multiple texts on different rows, you should specify your rows by 16 to avoid text overlapping.
​​
oled.show()
​
Lastly, we'll use the show() method from the 'oled' object to successfully display the messages on the OLED display.