Arduino with Ultrasonic sensor
Ultrasonic sensor (HC-SRO4)
As the name implies, ultrasonic sensors measure distance using ultrasonic waves. The sensor transmitter emits an ultrasonic wave which bounces off the target object to the reciever. Ultrasonic sensors measure the distance to the target by measuring the time between emission and reception. We use the HC-SR04 Ultrasonic module to work with Arduino. The HC-SR04 provides 2cm-400cm non-contact measurement function. It is inexpensive and easy to use, also has an available library to make integration much easier.
Below, we will look at how to measure distance from object placed in front of the HC-SR04 Ultrasonic sensor and display the result in real-time using Serial monitor.
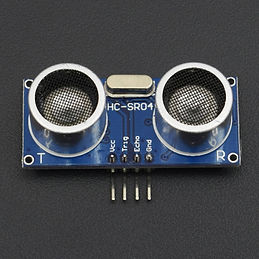
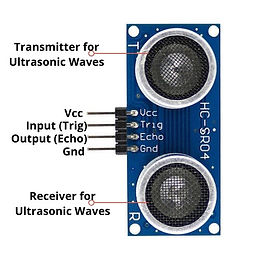
Schematic
​
Required components
-
Arduino UNO R3
-
HC-SR04 Ultrasonic sensor
-
Solderless breadboard
-
Jumper wires
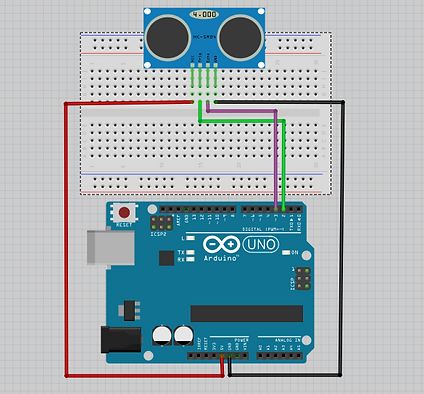
Sketch
​
const int TrigPin = 2;//Trig attach to pin2
const int EchoPin = 3;//Echo attach to pin3
float cm;
void setup() {
pinMode(TrigPin,OUTPUT);
pinMode(EchoPin,INPUT);
Serial.begin(9600);
}
void loop(){
digitalWrite(TrigPin,LOW);
delayMicroseconds(2);
digitalWrite(TrigPin,HIGH);
delayMicroseconds(10);
digitalWrite(TrigPin,LOW);
cm = pulseIn(EchoPin,HIGH)/58.0;
cm = (int(cm * 100.0))/100.0;
Serial.print("Distance: ");
Serial.print(cm);
Serial.println(" cm");
}
The above project will measure distance from object placed in line-of-sight of the HC-SR04 sensor and display the result in real-time using Serial monitor.
The ultrasonic sensor is great for of projects involving distance measurements or obstacle detection for example.