Arduino with LCD
LCD modules are commonly used in most embedded projects, reason being its cheap price, availability and friendly for makers and hobbyists. Here we will be using LCD1602 alphanumeric LCD display module as our feedback display device. This module can be used to display characters (alphabets), numbers, and custom generated characters such as symbols. Its consists of two rows and each can print 16 characters. Each character is built by a 5x8 pixel box. They are available in green and blue backlight, and operating voltage is 4.7 to 5.3v.

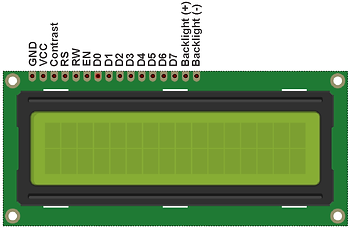
LCD display
Here we will learn how to wire our LCD display to the Arduino an write a simple sketch to display "Hello, World!" on our display.
Schematic
​
The LCD needs six Arduino digital output pins, and 5V and GND connections. A
potentiometer is also needed to control the contrast of the display.

Sketch
​
// include the library code:
#include <LiquidCrystal.h>
​
// initialize the library with the numbers of the interface pins
LiquidCrystal lcd(7, 8, 9, 10, 11, 12);
​
void setup() {
// set up the LCD's number of columns and rows:
lcd.begin(16, 2);
// Print a message to the LCD.
lcd.print("Hello, World!");
}
​
void loop() {
// set the cursor to column 0, line 1
// (note: line 1 is the second row, since counting begins with 0):
lcd.setCursor(0, 1);
}