Arduino with Keypad
Keypad (Membrane switch module)
Keypads are a great input device for interacting with your projects. In this tutorial we'll be using the 4x4 matrix membrane switch keypad with Arduino, although we will also look at how to interface the 3x4 matrix keypad.

The buttons on the keypads are arranged in rows and columns. A 3x4 keypad has 4 rows and 3 columns, and a 4x4 matrix keypad has 4 row and 4 columns. (the 4x4 keypad is a 3x4 keypad with additional column containing letter A-D.) Under each key is a membrane switch. All the switches in a row are connected together, same with the switches in a column using a conductive trace. Each row and column is brought out to a single pin, for a total of 8 pins in the case of a 4x4 matrix keypad.
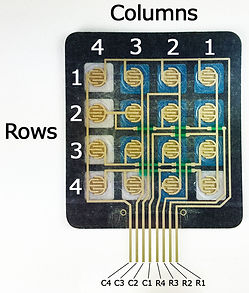
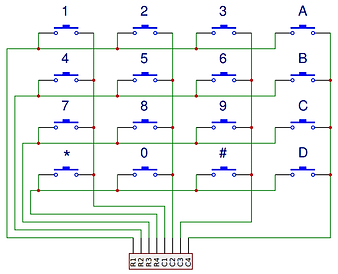
The Arduino is used to detect which button is pressed by detecting the row and column pin that's connected to the Arduino. Below, we will interface a 4x4 and 3x4 membrane switch modules to the Arduino and program it to show key presses on the Arduino IDE Serial monitor.
Schematic
​
Required components.
-
Arduino UNO R3.
-
4x4 Membrane switch module.
-
Jumper wires.
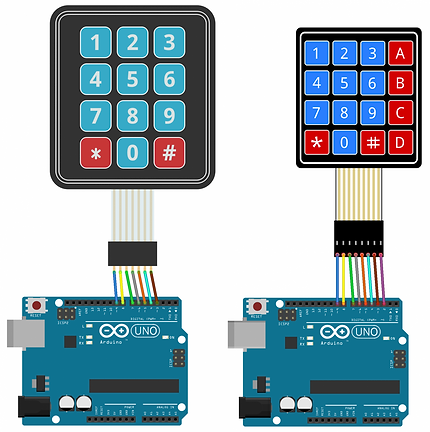
Sketch
​
//Sketch for a 4x4 membrane switch keypad.
​
#include <Keypad.h>
const byte ROWS = 4; //four rows
const byte COLS = 4; //four columns
​
//define the two-dimensional array on the buttons of the keypads
char hexaKeys[ROWS][COLS] = {
{'1','2','3','A'},
{'4','5','6','B'},
{'7','8','9','C'},
{'*','0','#','D'}
};
​
byte rowPins[ROWS] = {9, 8, 7, 6}; //connect to the row pinouts of the keypad
byte colPins[COLS] = {5, 4, 3, 2}; //connect to the column pinouts of the keypad
​
//initialize an instance of class NewKeypad
Keypad customKeypad = Keypad( makeKeymap(hexaKeys), rowPins, colPins, ROWS, COLS);
​
void setup(){
Serial.begin(9600);
}
void loop(){
char customKey = customKeypad.getKey();
if (customKey){
Serial.println(customKey);
}
}
​
**********************************************************
​
//Sketch for a 3x4 membrane switch keypad
​
#include <Keypad.h>
const byte ROWS = 4; //four rows
const byte COLS = 3; //three columns
​
//define the two-dimensional array on the buttons of the keypads
char hexaKeys[ROWS][COLS] = {
{'1','2','3'},
{'4','5','6'},
{'7','8','9'},
{'*','0','#'}
};
​
byte rowPins[ROWS] = {9, 8, 7, 6}; //connect to the row pinouts of the keypad
byte colPins[COLS] = {5, 4, 3, 2}; //connect to the column pinouts of the keypad
​
//initialize an instance of class NewKeypad
Keypad customKeypad = Keypad( makeKeymap(hexaKeys), rowPins, colPins, ROWS, COLS);
​
void setup(){
Serial.begin(9600);
}
void loop(){
char customKey = customKeypad.getKey();
if (customKey){
Serial.println(customKey);
}
}